Repetition
Repetition, or widely known as looping, are a way to run a block of program again and again until a certain condition has been fullfilled.
These are the types of repetition used in C programming:
-) for loop :
These are the types of repetition used in C programming:
-) for loop :
The syntax of for loop is:
for (initializationStatement; testExpression; updateStatement) { // codes }
The initialization statement is executed only once.
Then, the test expression is evaluated. If the test expression is false (0), for loop is terminated. But if the test expression is true (nonzero), codes inside the body of
for
loop is executed and the update expression is updated.
This process repeats until the test expression is false.
The for loop is commonly used when the number of iterations is known.
for loop flowchart:
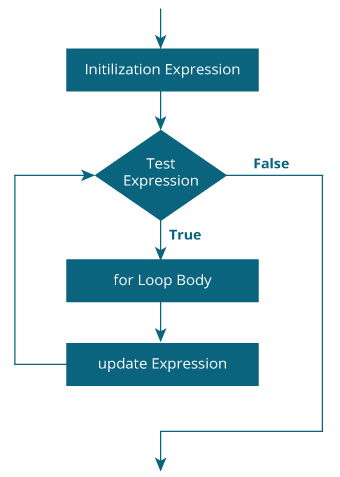
for loop example:
// Program to calculate the sum of first n natural numbers
// Positive integers 1,2,3...n are known as natural numbers
#include <stdio.h>
int main()
{
int num, count, sum = 0;
printf("Enter a positive integer: ");
scanf("%d", &num);
// for loop terminates when n is less than count
for(count = 1; count <= num; ++count)
{
sum += count;
}
printf("Sum = %d", sum);
return 0;
}
Output
Enter a positive integer: 10 Sum = 55
The value entered by the user is stored in variable num. Suppose, the user entered 10.
The count is initialized to 1 and the test expression is evaluated. Since, the test expression
count <= num
(1 less than or equal to 10) is true, the body of for loop is executed and the value of sum will equal to 1.
Then, the update statement
++count
is executed and count will equal to 2. Again, the test expression is evaluated. Since, 2 is also less than 10, the test expression is evaluated to true and the body of for loop is executed. Now, the sum will equal 3.
This process goes on and the sum is calculated until the count reaches 11.
When the count is 11, the test expression is evaluated to 0 (false) as 11 is not less than or equal to 10. Therefore, the loop terminates and next, the total sum is printed.
-) WHILE loop:
The syntax of a while loop is:
while (testExpression) { //codes }
where,
testExpression
checks the condition is true or false before each loop.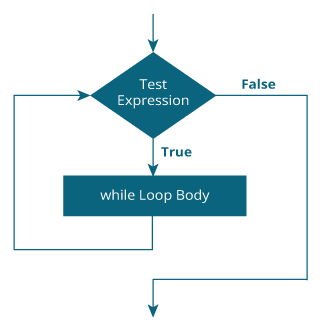
WHILE loop example:
// Program to find factorial of a number
// For a positive integer n, factorial = 1*2*3...n
#include <stdio.h>
int main()
{
int number;
long long factorial;
printf("Enter an integer: ");
scanf("%d",&number);
factorial = 1;
// loop terminates when number is less than or equal to 0
while (number > 0)
{
factorial *= number; // factorial = factorial*number;
--number;
}
printf("Factorial= %lld", factorial);
return 0;
}
Output
Enter an integer: 5 Factorial = 120
-) DO....WHILE loop:
is similar to WHILE loop, but with an important difference. The body of
do...while
loop is executed once, before checking the test expression. Hence, the do...while loop
is executed at least once.
the syntax for DO....WHILE loop is:
do { // codes } while (testExpression);
DO...WHILE flowchart:
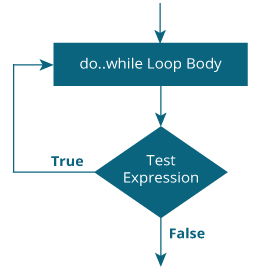
DO...WHILE loop example:
// Program to add numbers until user enters zero
#include <stdio.h>
int main()
{
double number, sum = 0;
// loop body is executed at least once
do
{
printf("Enter a number: ");
scanf("%lf", &number);
sum += number;
}
while(number != 0.0);
printf("Sum = %.2lf",sum);
return 0;
}
Output
Enter a number: 1.5 Enter a number: 2.4 Enter a number: -3.4 Enter a number: 4.2 Enter a number: 0 Sum = 4.70
Comments
Post a Comment