C Programming Functions
C Programming Functions
A function is a block of code that performs a specific task.
Suppose, a program related to graphics needs to create a circle and color it depending upon the radius and color from the user. You can create two functions to solve this problem:
- create a circle function
- color function
Dividing complex problem into small components makes program easy to understand and use.
Types of function
Depending on whether a function is defined by the user or already included in C compilers, there are two types of functions in C programming
There are two types of function in C programming:
- Standard library functions
- User defined functions
Standard library functions
The standard library functions are built-in functions in C programming to handle tasks such as mathematical computations, I/O processing, string handling etc.
These functions are defined in the header file. When you include the header file, these functions are available for use. For example:
The
printf()
is a standard library function to send formatted output to the screen (display output on the screen). This function is defined in "stdio.h"
header file.
There are other numerous library functions defined under
"stdio.h"
, such as scanf()
, fprintf()
, getchar()
etc. Once you include "stdio.h"
in your program, all these functions are available for use.User-defined function
As mentioned earlier, C allow programmers to define functions. Such functions created by the user are called user-defined functions.
You can create as many user-defined functions as you want.
How user-defined function works?
#include <stdio.h> void functionName() { ... .. ... ... .. ... } int main() { ... .. ... ... .. ... functionName(); ... .. ... ... .. ... }
The execution of a C program begins from the
main()
function.
When the compiler encounters
functionName();
inside the main function, control of the program jumps tovoid functionName()
And, the compiler starts executing the codes inside the user-defined function.
The control of the program jumps to statement next to
functionName();
once all the codes inside the function definition are executed.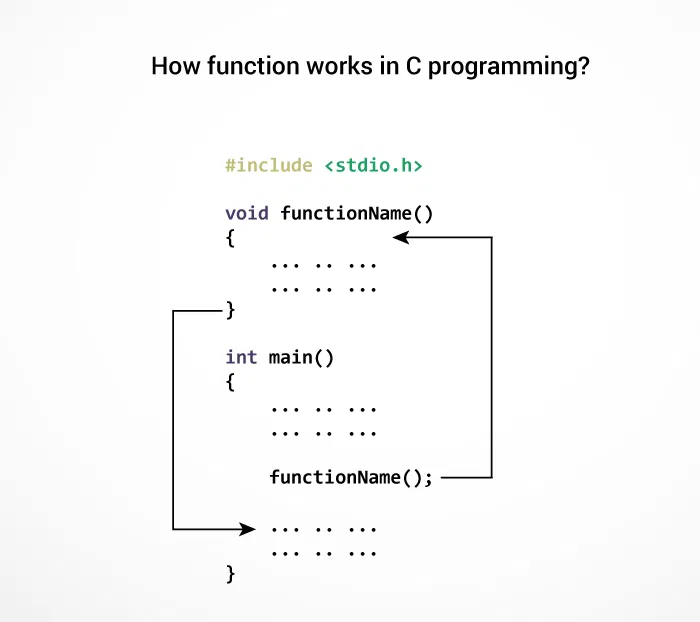
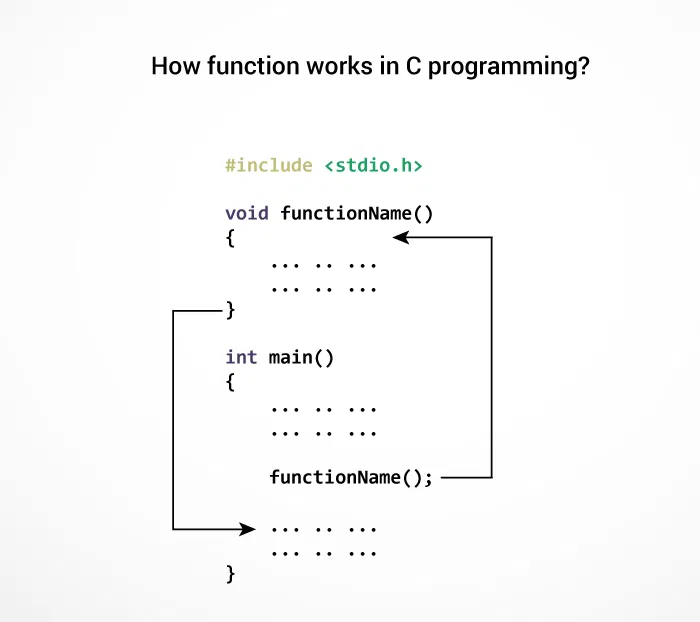
User-defined function
Here is an example to add two integers. To perform this task, an user-defined function
addNumbers()
is defined.#include <stdio.h>
int addNumbers(int a, int b); // function prototype
int main()
{
int n1,n2,sum;
printf("Enters two numbers: ");
scanf("%d %d",&n1,&n2);
sum = addNumbers(n1, n2); // function call
printf("sum = %d",sum);
return 0;
}
int addNumbers(int a,int b) // function definition
{
int result;
result = a+b;
return result; // return statement
}
Function prototype
A function prototype is simply the declaration of a function that specifies function's name, parameters and return type. It doesn't contain function body.
A function prototype gives information to the compiler that the function may later be used in the program.
Syntax of function prototype
returnType functionName(type1 argument1, type2 argument2,...);
In the above example,
int addNumbers(int a, int b);
is the function prototype which provides following information to the compiler:- name of the function is
addNumbers()
- return type of the function is
int
- two arguments of type
int
are passed to the function
The function prototype is not needed if the user-defined function is defined before the
main()
function.Calling a function
Control of the program is transferred to the user-defined function by calling it.
Syntax of function call
functionName(argument1, argument2, ...);
In the above example, function call is made using
addNumbers(n1,n2);
statement inside the main()
.Function definition
Function definition contains the block of code to perform a specific task i.e. in this case, adding two numbers and returning it.
Syntax of function definition
returnType functionName(type1 argument1, type2 argument2, ...) { //body of the function }
When a function is called, the control of the program is transferred to the function definition. And, the compiler starts executing the codes inside the body of a function.
Passing arguments to a function
In programming, argument refers to the variable passed to the function. In the above example, two variables n1 and n2 are passed during function call.
The parameters a and b accepts the passed arguments in the function definition. These arguments are called formal parameters of the function.
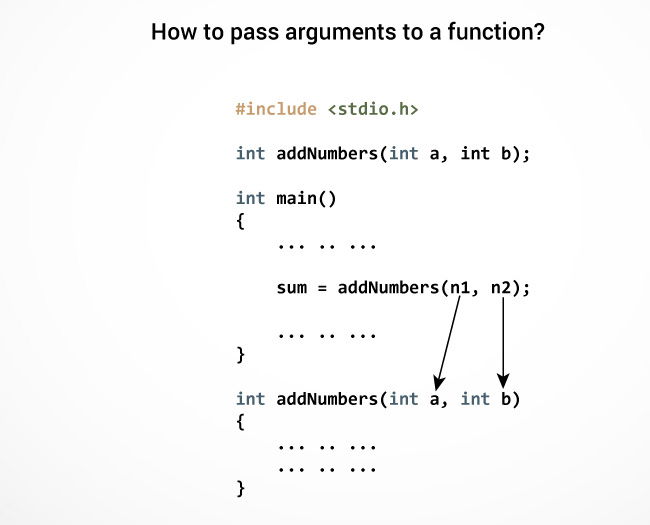
The type of arguments passed to a function and the formal parameters must match, otherwise the compiler throws error.
If n1 is of char type, a also should be of char type. If n2 is of float type, variable b also should be of float type.
A function can also be called without passing an argument.
Return Statement
The return statement terminates the execution of a function and returns a value to the calling function. The program control is transferred to the calling function after return statement.
In the above example, the value of variable result is returned to the variable sum in the
main()
function.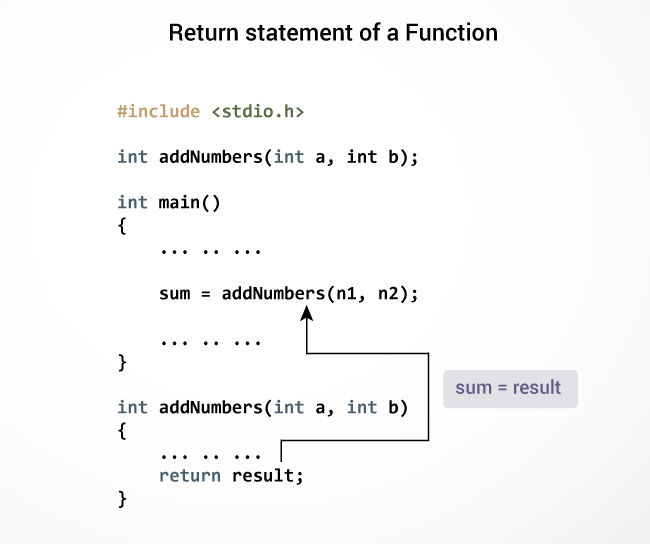
Syntax of return statement
return (expression);
For example,
return a; return (a+b);
The type of value returned from the function and the return type specified in function prototype and function definition must match.
These 4 programs below check whether the integer entered by the user is prime number or not. The output of all these programs below is same, and we have created a user-defined function in each example. However, the approch we have taken in each example is different.
Example 1: No arguments passed and no return Value
#include <stdio.h>
void checkPrimeNumber();
int main()
{
checkPrimeNumber(); // argument is not passed
return 0;
}
// return type of the function is void because function is not returning anything
void checkPrimeNumber()
{
int n, i, flag=0;
printf("Enter a positive integer: ");
scanf("%d",&n);
for(i=2; i <= n/2; ++i)
{
if(n%i == 0)
{
flag = 1;
}
}
if (flag == 1)
printf("%d is not a prime number.", n);
else
printf("%d is a prime number.", n);
}
The
checkPrimeNumber()
function takes input from the user, checks whether it is a prime number or not and displays it on the screen.
The empty parentheses in
checkPrimeNumber();
statement inside the main()
function indicates that no argument is passed to the function.
The return type of the function is
void
. Hence, no value is returned from the function.Example 2: No arguments passed but a return value
#include <stdio.h>
int getInteger();
int main()
{
int n, i, flag = 0;
n = getInteger(); // no argument is passed
for(i=2; i<=n/2; ++i)
{
if(n%i==0){
flag = 1;
break;
}
}
if (flag == 1)
printf("%d is not a prime number.", n);
else
printf("%d is a prime number.", n);
return 0;
}
int getInteger() // returns integer entered by the user
{
int n;
printf("Enter a positive integer: ");
scanf("%d",&n);
return n;
}
The empty parentheses in
n = getInteger();
statement indicates that no argument is passed to the function. And, the value returned from the function is assigned to n.
Here, the
getInteger()
function takes input from the user and returns it. The code to check whether a number is prime or not is inside the main()
function.Example 3: Argument passed but no return value
#include <stdio.h>
void checkPrimeAndDisplay(int n);
int main()
{
int n;
printf("Enter a positive integer: ");
scanf("%d",&n);
// n is passed to the function
checkPrimeAndDisplay(n);
return 0;
}
// void indicates that no value is returned from the function
void checkPrimeAndDisplay(int n)
{
int i, flag = 0;
for(i=2; i <= n/2; ++i)
{
if(n%i == 0){
flag = 1;
break;
}
}
if(flag == 1)
printf("%d is not a prime number.",n);
else
printf("%d is a prime number.", n);
}
The integer value entered by the user is passed to
checkPrimeAndDisplay()
function.
Here, the
checkPrimeAndDisplay()
function checks whether the argument passed is a prime number or not and displays the appropriate message.Example 4: Argument passed and a return value
#include <stdio.h>
int checkPrimeNumber(int n);
int main()
{
int n, flag;
printf("Enter a positive integer: ");
scanf("%d",&n);
// n is passed to the checkPrimeNumber() function
// the value returned from the function is assigned to flag variable
flag = checkPrimeNumber(n);
if(flag == 1)
printf("%d is not a prime number",n);
else
printf("%d is a prime number",n);
return 0;
}
// integer is returned from the function
int checkPrimeNumber(int n)
{
int i;
for(i=2; i <= n/2; ++i)
{
if(n%i == 0)
return 1;
}
return 0;
}
The input from the user is passed to
checkPrimeNumber()
function.
The
checkPrimeNumber()
function checks whether the passed argument is prime or not. If the passed argument is a prime number, the function returns 0. If the passed argument is a non-prime number, the function returns 1. The return value is assigned to the flag variable.
Depending on whether flag is 0 or 1, appropriate message is printed from the
main()
function.Which approach is better?
Well, it depends on the problem you are trying to solve. In this case, passing argument and returning a value from the function (example 4) is better.
A function should perform a specific task. The
checkPrimeNumber()
function doesn't take input from the user nor it displays the appropriate message. It only checks whether a number is prime or not, which makes code your code easy to understand and debug.
Comments
Post a Comment